Blog / Build your ecommerce store with Next.js Commerce and Swell on Next 13
Build your ecommerce store with Next.js Commerce and Swell on Next 13
Discover the simple steps to build and launch an ecommerce website by leveraging Next.js, Swell, and Next.js Commerce.
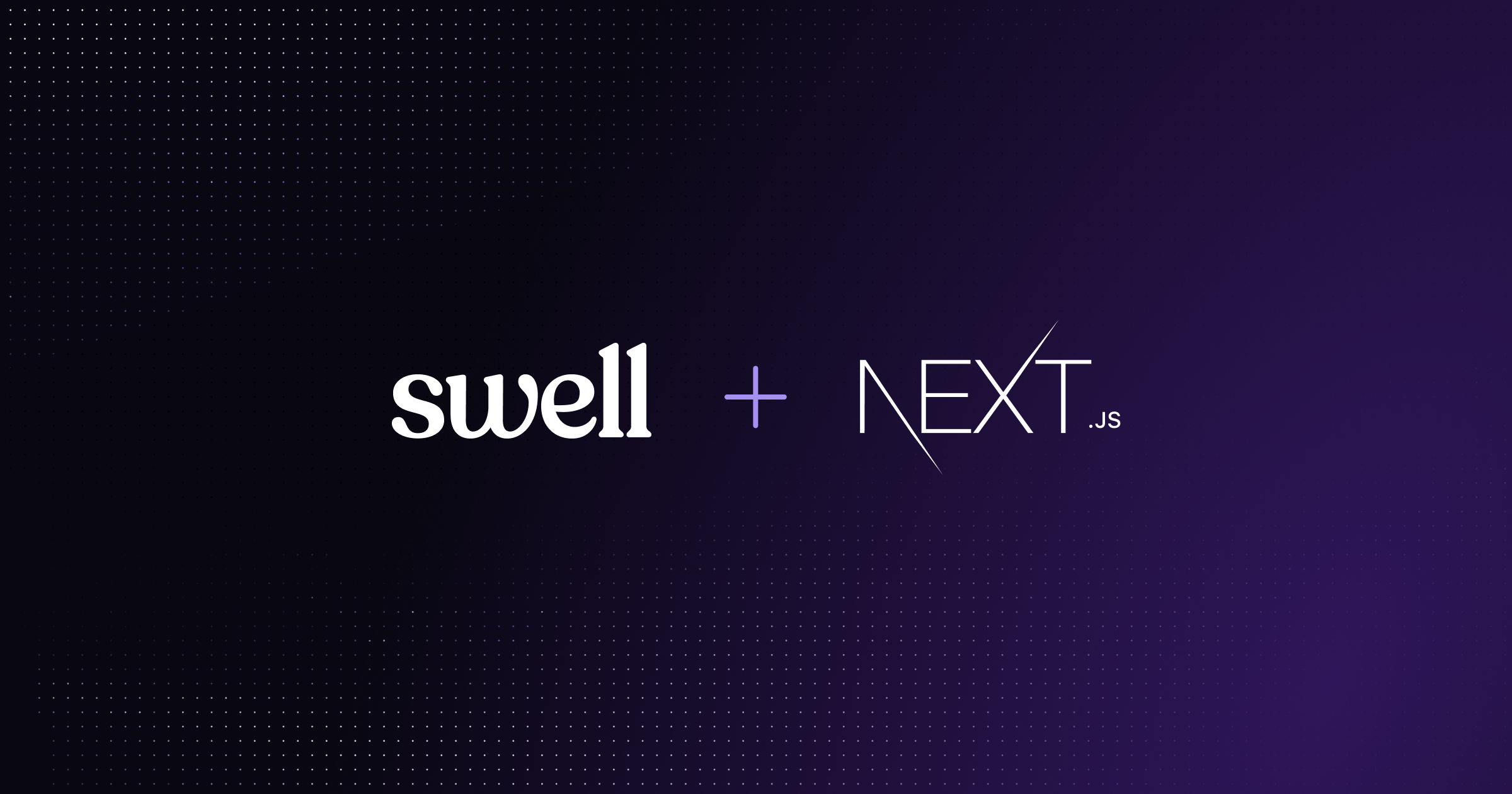
This guide provides an overview of how to quickly create high-performance ecommerce websites on Swell using Next.js Commerce. With this starter kit, you can clone, customize, and deploy your own store in just a few clicks.
And for more, be sure to watch this tutorial by Hamed Bahram of Monogram on the same topic: Build an e-commerce store using NextJs 13 and Swell.
Next.js Commerce
Next.js Commerce offers a range of benefits, such as image optimization rendering, internationalization, and analytics reporting. It also includes advanced features like incremental static generation, eliminating the need to manual trigger webhooks. It also simplifies the development process by providing a fully styled UI components for core ecommerce functionality, and automatically generating default settings for Swell.
This guide uses Vercel as a hosting solution—Vercel offers hassle-free asset serving through the CDN and seamless integration with Next.js' automatic static optimization and API routes.
Getting started
First, create a store in Swell. Hit this link and follow the signup steps.
Once you’ve created an account, you’ll need to deploy Next-Commerce directly from Vercel.
Clone and Deploy
1. Click this magic button to deploy the starter kit directly to Vercel.
2. Create a git repository on Github, Gitlab, or Bitbucket. You might need to set up further permissions if prompted.
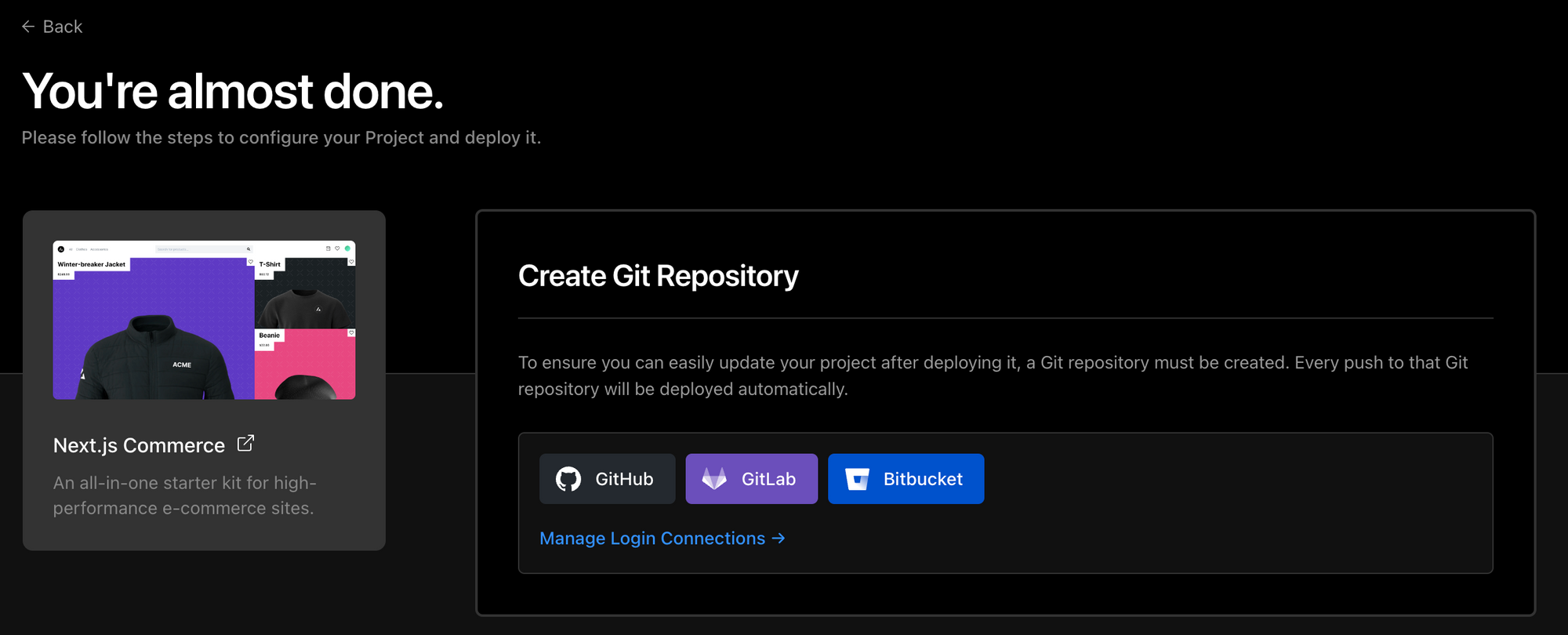
3. Give your new repository a name, and click Create.
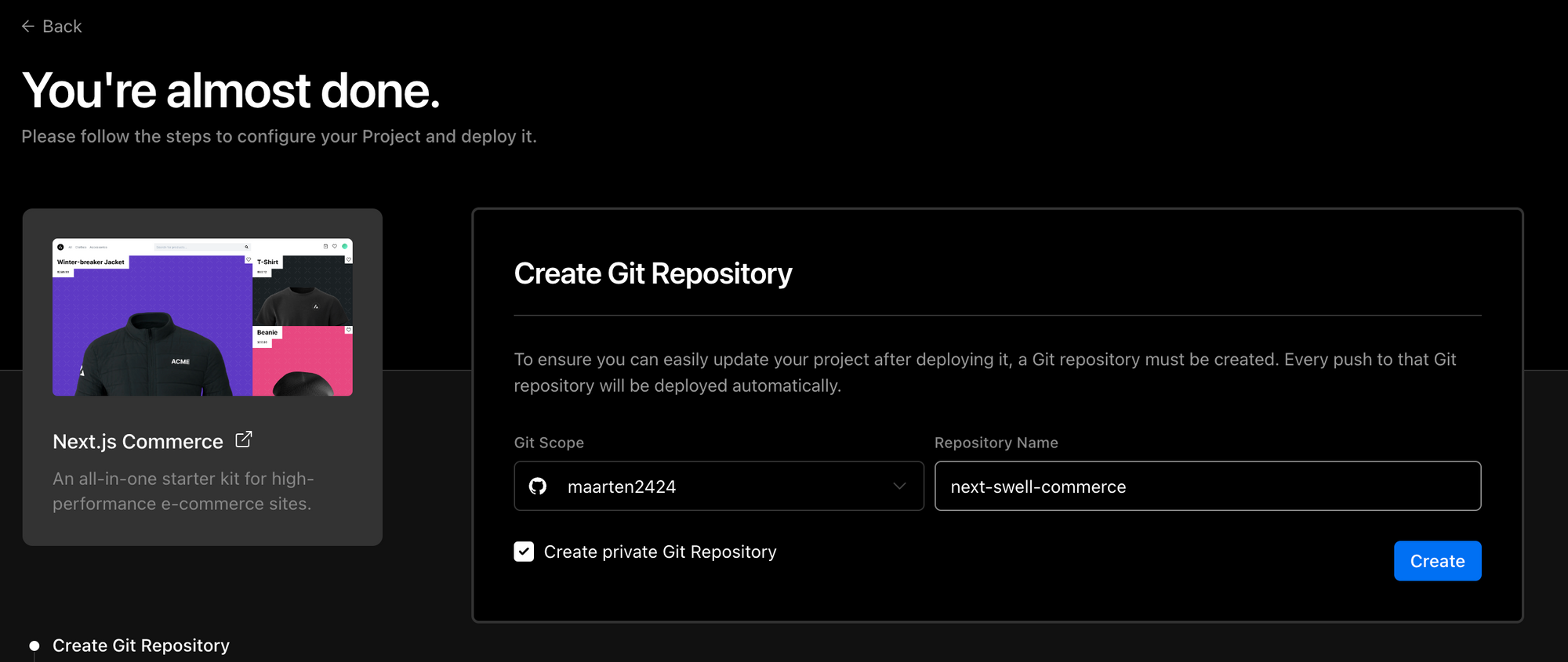
4. In the Add Integrations step, click Add to connect Swell to your project.
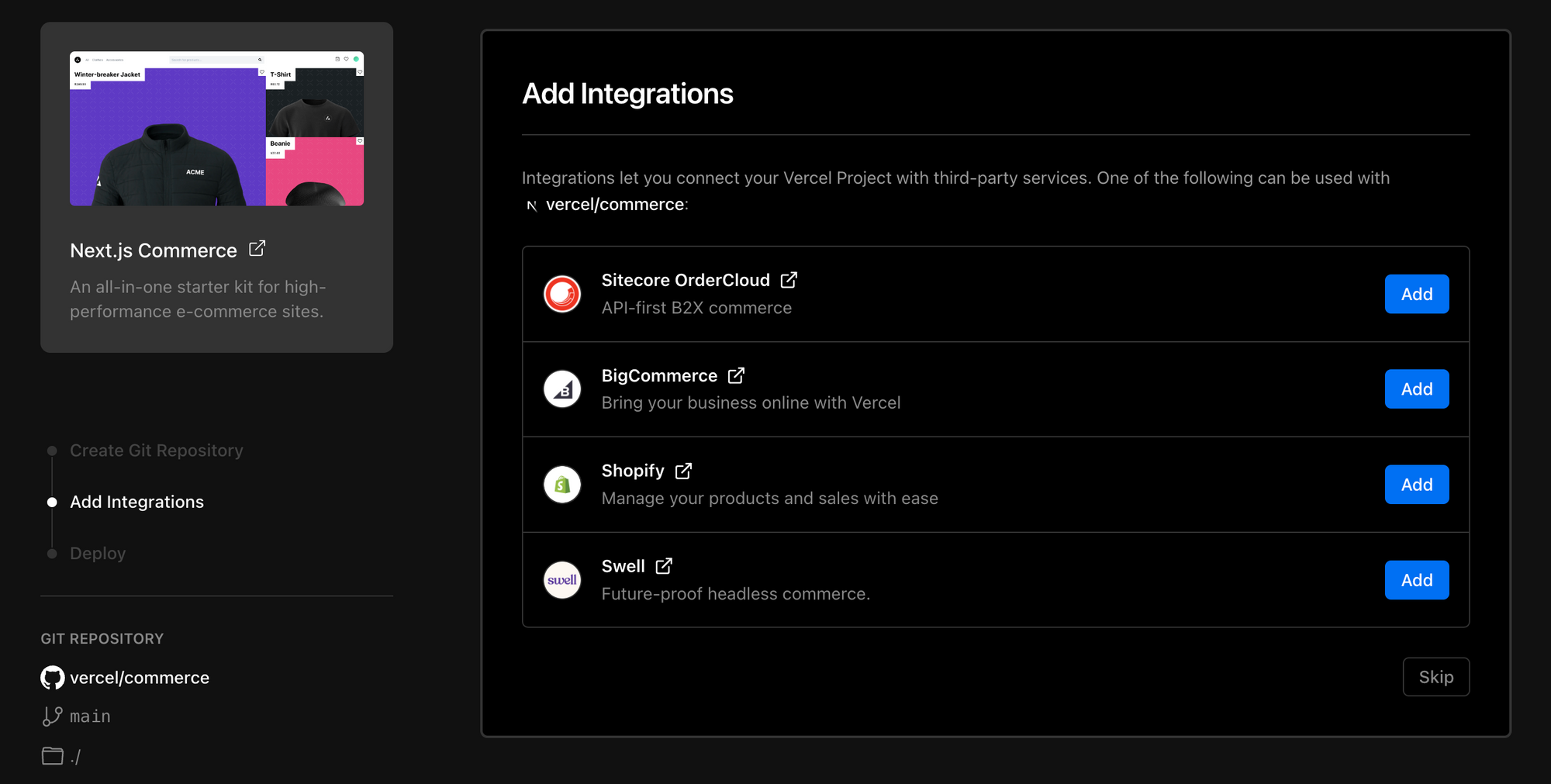
5. Click Connect to an existing store. Alternatively, you can also create a new account.
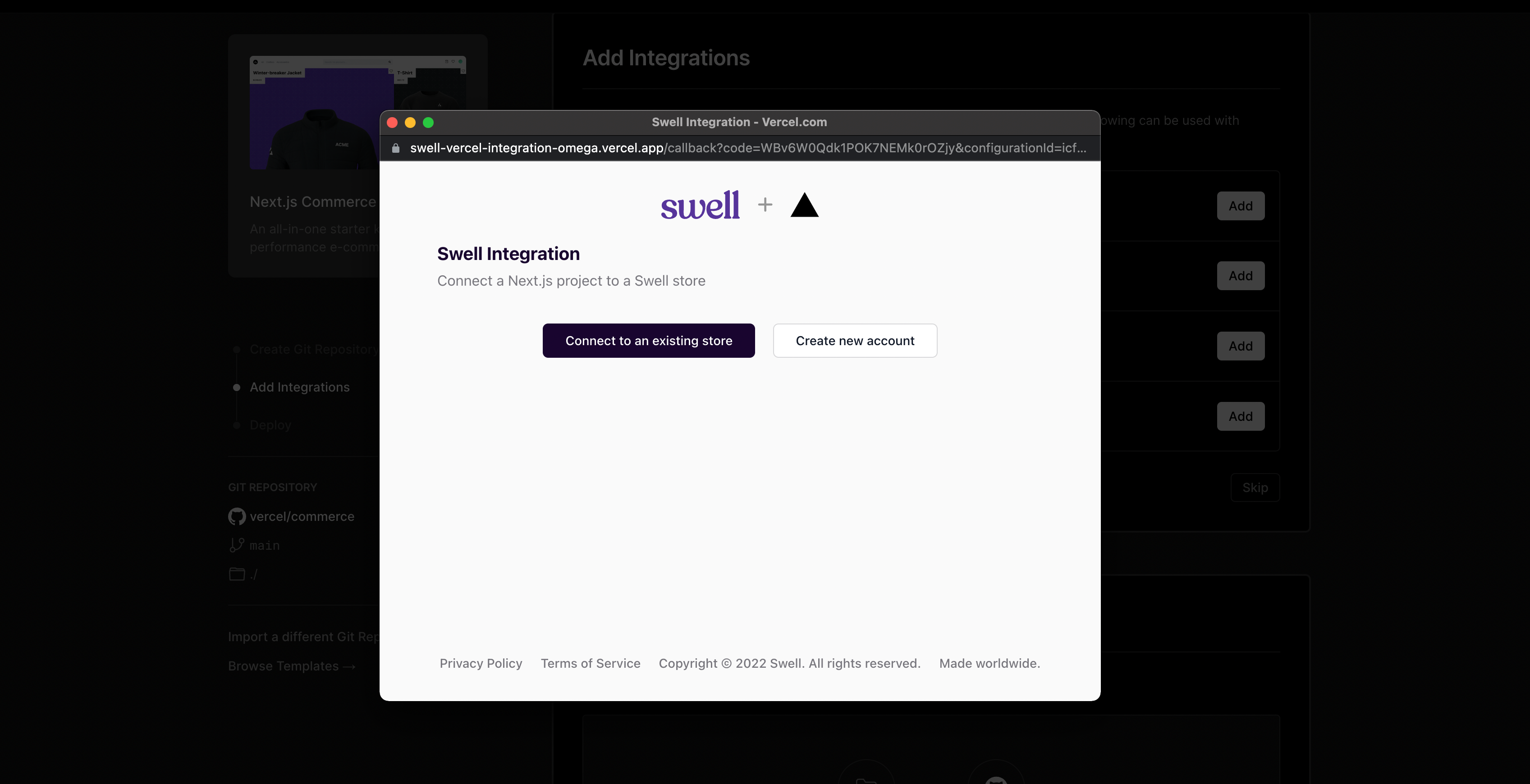
6. Insert your Swell store domain, and hit Authorize integration.
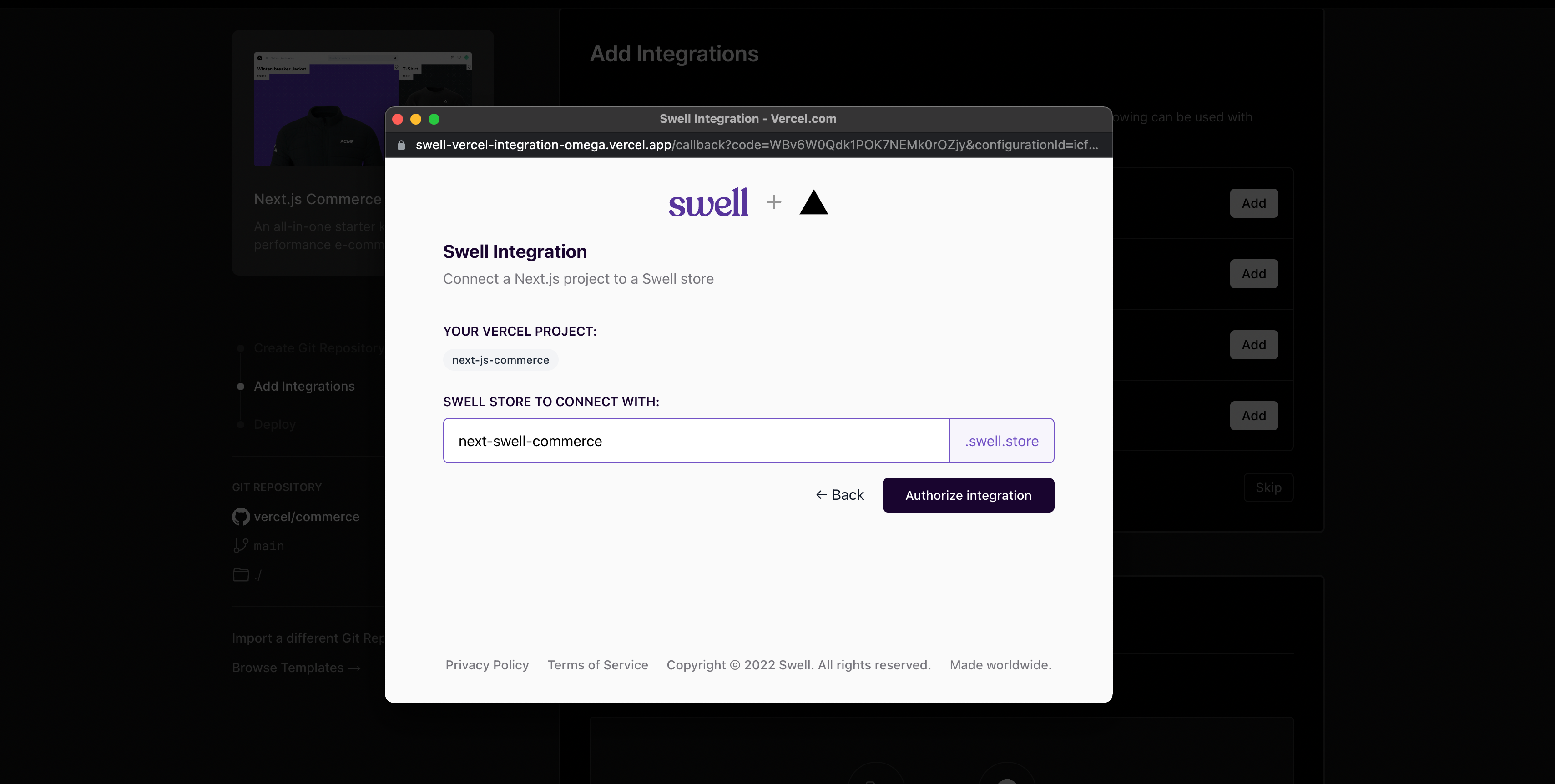
7. By clicking Allow, you grant Vercel access to all the necessary information required to connect with your store. This prompt will display everything that is needed for this connection.
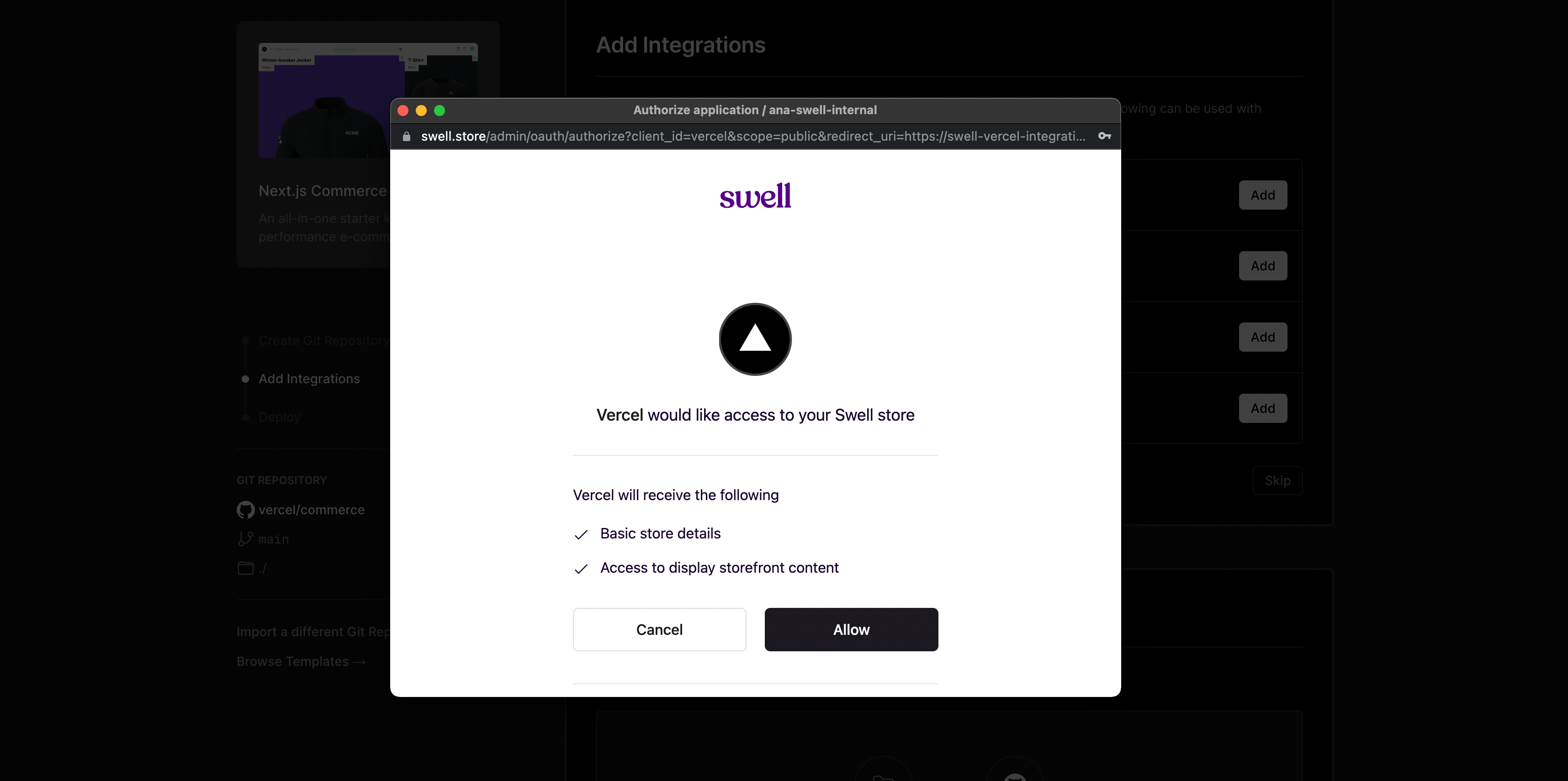
8. You’re done! 🎉 You’ve successfully deployed your project on Vercel.
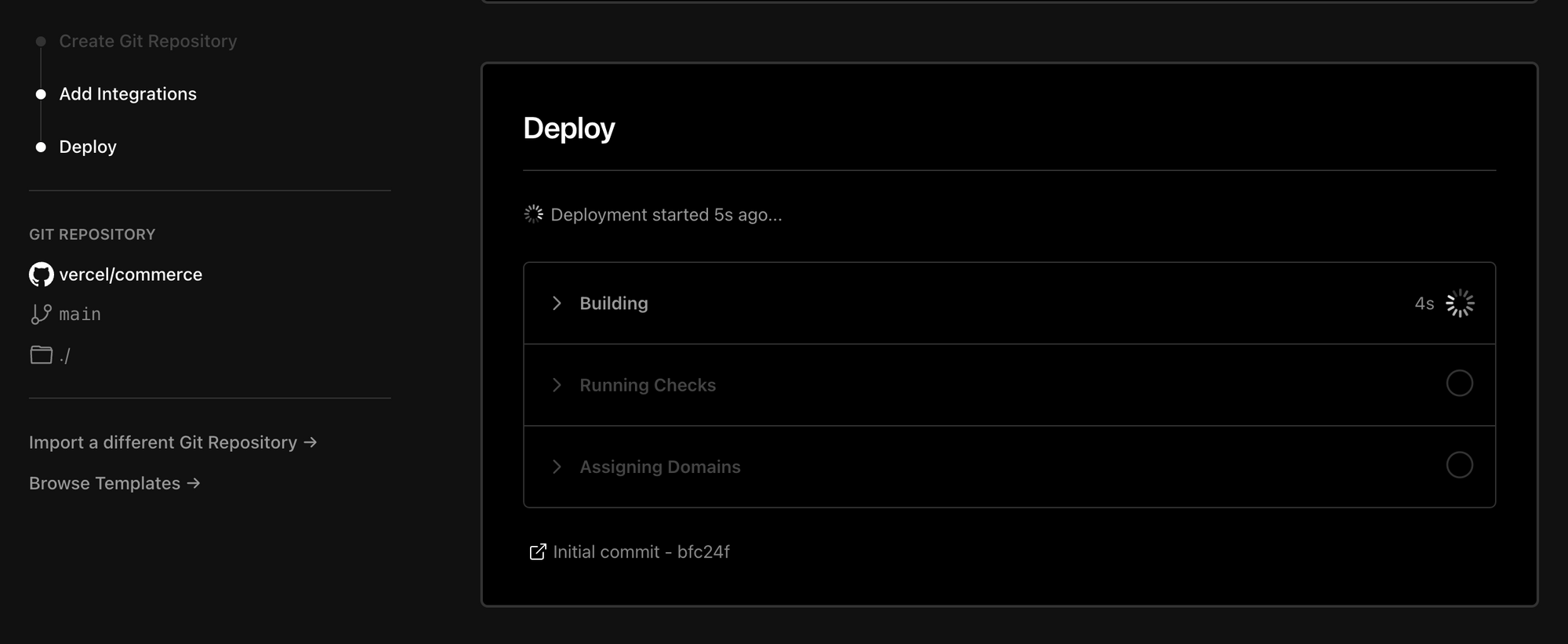
Running the project locally
To customize your store, you’ll need to make some changes locally. Make sure you’re running git and node.js in your system. Next-Commerce uses pnpm as a package manager — which is up to 2x faster than yarn or npm and more efficient.
In the repository you’ll find a monorepo with the following directory structure:
- packages/
- commerce/: This is the base definition to serve for all the providers.
- swell/: The provider for Swell.
- site/: Next.js website.
- components/ : Shared components
- pages/ : Individual pages
- assets/ : CSS styling configuration
Clone the repository and run this command to install:
$ pnpm install & pnpm build
Once you have cloned the project, you’ll need to create a .env file based on the .env.template provided within the project. The file requires your Swell Store ID and your public API key — both of which can be found in the Swell dashboard under Developer > API Keys.
In the .env, provide your Swell Store ID and public API key in the following format:
COMMERCE_PROVIDER=@vercel/commerce-swell
NEXT_PUBLIC_SWELL_STORE_ID=XXXXXXX
NEXT_PUBLIC_SWELL_PUBLIC_KEY=XXXXXXX
With the .env file configured with your Swell store information, you’re ready to deploy your storefront. Fill out any additional settings or configurations, and you're ready to deploy on your hosting provider of choice.
And to run the dev server:
$ pnpm dev
Customization
After setting up Next.js Commerce and Swell, you have all the necessary information to begin building a complete ecommerce storefront with all the required UI elements. The next step is to personalize and tailor it to their specific needs.
Navigation
To begin, set your own custom logo. You can set it by customizing Logo.tsx
This will set a custom logo on every part of your storefront.
const Logo = ({ className = '', ...props }) => (
<svg
width="32"
height="32"
viewBox="0 0 32 32"
fill="none"
xmlns="http://www.w3.org/2000/svg"
className={className}
{...props}
>
// some custom SVG
</svg>
)
export default Logo
Footer
The footer of the storefront is still using its demo data. Here’s how to quickly change that.
const year = () => {
const date = new Date()
return date.getFullYear()
}
<div className="pt-6 pb-10 flex flex-col md:flex-row justify-between items-center space-y-4 text-accent-6 text-sm">
<div>
<span>© {year()} Swell, Inc. All rights reserved.</span>
</div>
</div>
Theme
To personalize the website, you can modify the Tailwind configuration. The storefront's appearance is created using TailwindCSS, a convenient CSS framework for rapidly developing interfaces. You can further customize the theme using the tailwind.config.js file.
// tailwind.config.js
primary: 'var(--primary)',
'primary-2': 'var(--primary-2)',
secondary: 'var(--secondary)',
'secondary-2': 'var(--secondary-2)',
hover: 'var(--hover)',
'hover-1': 'var(--hover-1)',
'hover-2': 'var(--hover-2)',
'accent-0': 'var(--accent-0)',
'accent-1': 'var(--accent-1)',
'accent-2': 'var(--accent-2)',
'accent-3': 'var(--accent-3)',
'accent-4': 'var(--accent-4)',
'accent-5': 'var(--accent-5)',
'accent-6': 'var(--accent-6)',
'accent-7': 'var(--accent-7)',
'accent-8': 'var(--accent-8)',
'accent-9': 'var(--accent-9)',
Next.js Commerce sets most of the theme settings from CSS Variables. These are being set in the /site/assets/base.css file:
:root {
--primary: #ffffff; // Primary Colors
--primary-2: #f1f3f5;
--secondary: #000000;
--secondary-2: #111;
--selection: var(--cyan);
--text-base: #000000;
--text-primary: #000000;
--text-secondary: white;
--hover: rgba(0, 0, 0, 0.075); // Hovers
--hover-1: rgba(0, 0, 0, 0.15);
--hover-2: rgba(0, 0, 0, 0.25);
--cyan: #22b8cf;
--green: #37b679;
--red: #da3c3c;
--purple: #f81ce5;
--blue: #0070f3;
--pink: #ff0080;
--pink-light: #ff379c;
--magenta: #eb367f;
--violet: #7928ca;
--violet-dark: #4c2889;
--accent-0: #fff; // Accent Colors
--accent-1: #fafafa;
--accent-2: #eaeaea;
--accent-3: #999999;
--accent-4: #888888;
--accent-5: #666666;
--accent-6: #444444;
--accent-7: #333333;
--accent-8: #111111;
--accent-9: #000;
--font-sans: -apple-system, system-ui, BlinkMacSystemFont, 'Helvetica Neue',
'Helvetica', sans-serif; // Set Fonts
}
Next.js Commerce also has a dark theme—you can set your own colors through the following:
[data-theme='dark'] {
--primary: #000000;
--primary-2: #111;
--secondary: #ffffff;
--secondary-2: #f1f3f5;
--hover: rgba(255, 255, 255, 0.075);
--hover-1: rgba(255, 255, 255, 0.15);
--hover-2: rgba(255, 255, 255, 0.25);
--selection: var(--purple);
--text-base: white;
--text-primary: white;
--text-secondary: black;
--accent-9: #fff;
--accent-8: #fafafa;
--accent-7: #eaeaea;
--accent-6: #999999;
--accent-5: #888888;
--accent-4: #666666;
--accent-3: #444444;
--accent-2: #333333;
--accent-1: #111111;
--accent-0: #000;
}
Conclusion
We have only just begun to explore all that Next.js Commerce has to offer. There is still a lot more for us to discover and develop with Swell and Next Commerce. We are actively striving to improve our own SDK to be used by many platforms and to include all the features that Swell offers.
Follow us on Twitter and get involved in our discussions for the latest updates.
Up next
right to your inbox