Blog / Swell + Next.JS + GraphQL: Building modern ecommerce experiences
Swell + Next.JS + GraphQL: Building modern ecommerce experiences
Explore how to use Swell's GraphQL API and TypeScript to build cutting-edge online stores with ease.
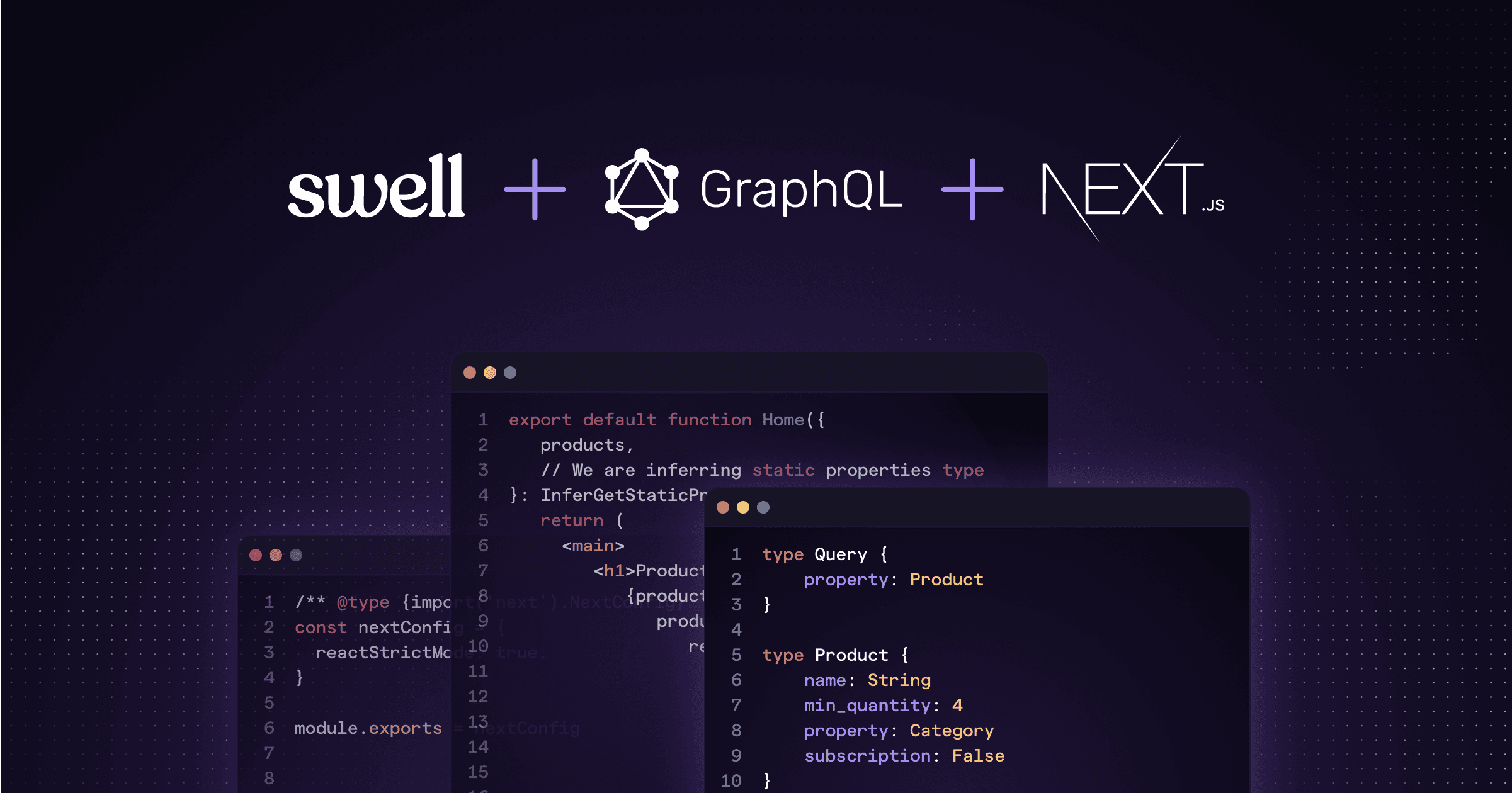
Flexibility and efficiency with GraphQL
One of the key advantages of using Swell for ecommerce is its GraphQL API, which allows you to retrieve precisely the data you need in a single request. This means no more over-fetching or under-fetching of data, resulting in more efficient and optimized queries and responses. It also provides introspection capabilities, allowing you to explore and understand the available data and operations, making development a breeze.
Swell's GraphQL API is designed to be flexible, allowing you to customize the data you retrieve based on your specific requirements. You can query for products, orders, customers, and more, with the ability to filter, sort, and paginate the results. Swell's GraphQL also supports mutations, enabling you to create, update, and delete data, providing complete CRUD (Create, Read, Update, Delete) functionality.
When properly set up with Visual Studio Code, you’ll get instant feedback while writing your queries. This can help you understand the main concepts of models on Swell, including custom fields. It provides GraphQL syntax highlighting and autocompletion: If you’ve installed the "GraphQL Language Feature Support" extension, Visual Studio Code will provide syntax highlighting and autocompletion for GraphQL queries, mutations, and types in your code. This can help you write valid GraphQL queries and mutations faster and with fewer errors.
Developer-friendly with TypeScript support
As a modern and statically-typed language, TypeScript has become increasingly popular among developers to build scalable and maintainable applications. Swell fully embraces TypeScript, providing comprehensive TypeScript support in its GraphQL API. Swell's GraphQL schema is fully typed, providing strong type-checking capabilities for both queries and mutations.
With TypeScript support, you can enjoy improved developer experience, including code completion, type-checking, and documentation within your IDE. This makes it easier to catch errors early in the development process, reducing bugs and improving the quality of your code. Swell's TypeScript support also helps with code refactoring, making it easier to navigate and maintain your codebase.
Installation
Setting up Swell, GraphQL, and TypeScript with Next.js is a straightforward process. See the steps below:
1. Create a Next.js project.
If you haven't already, create a new Next.js project using the Next.js boilerplate. Make sure you select typescript support:
npx create-next-app@latest --typescript
2. Install dependencies.
Install the necessary dependencies for GraphQL and Typescript generation in your Next.js project. You'll need a GraphQL client library (we’re using graphql-request), and you’ll need to install graphql-codegen to generate types.
npm i --save-dev graphql-codegen @graphql-codegen/typescript-graphql-request graphql-request
3. Create a .graphqlrc.yml configuration file.
This enables easy previewing of GraphQL queries directly in Visual Studio Code.
schema:
- https://[your_store_name].swell.store/graphql:
headers:
Authorization: "[your_public_key]"
documents: src/**/*.{graphql,gql,js,ts,jsx,tsx}
4. Install the GraphQL: Language Feature Support plugin.
5. Set up GraphQL Code Generator to generate the correct types.
import { CodegenConfig } from "@graphql-codegen/cli";
const config: CodegenConfig = {
schema: {
"https://[your_store_name].swell.store/graphql": {
headers: {
Authorization: "[your_public_key]",
},
},
},
documents: ["src/**/*.gql"],
generates: {
"./src/__generated__/sdk.ts": {
plugins: [
"typescript",
"typescript-operations",
"typescript-graphql-request",
],
config: {
rawRequest: true, // includes data and errors
preResolveTypes: false, // don't preresolve types so descriptions are loaded
},
},
},
};
export default config;
6. Add the graphql-codegen watch function to your package.json dev command.
This will start graphql-codegen alongside next dev, creating and updating the typescript types automatically.
"dev": "next dev & graphql-codegen -w",
7. Create a Swell graphql-request client with the generated SDK.
import { GraphQLClient } from "graphql-request";
import { getSdk } from "@/__generated__/sdk";
const client = new GraphQLClient("https://[your_store_name].swell.store/graphql", {
headers: {
Authorization: "[your_public_key]",
},
});
export default getSdk(client);
8. Create your queries.
We’ve set up graphql-codegen so it creates an SDK based on your queries. This is useful for creating inline documentation with our API. For this example, we’ll store our queries in the src/queries/[query].gql folder. With this setup, you also get autocompletion, making writing queries even easier.
query getAllProducts {
products {
count
results {
name
id
options {
price
}
images {
file {
url
}
}
}
}
}
9. Happy developing!
Graphql-codegen generates a fully typed SDK that you can use on individual pages (or anywhere). Here’s an example of implementing the getProductBySlug query on a single page.
import { InferGetStaticPropsType } from "next";
import client from "~/lib/client";
export const getStaticProps = async () => {
// Client returns our query as SDK format
const { data } = await client.getAllProducts();
return {
props: {
products: data.products?.results,
},
};
};
export default function Home({
products,
// We are infering static properties type
}: InferGetStaticPropsType<typeof getStaticProps>) {
return (
<main>
<h1>Products</h1>
{products ? (
products.map((product) => {
return (
<div key={product?.id}>
<h2>{product?.name}</h2>
<p>{product?.description}</p>
<p>{product?.slug}</p>
</div>
);
})
) : (
<p>No products found</p>
)}
</main>
);
}
As you can see, there’s no need to manually import specific types. Everything is directly inferred by our SDK, and reflects your specific store’s API including your custom models and fields!
Start building modern commerce experiences
By combining Swell, Next.js, and GraphQL, developers can create modern ecommerce experiences that are both flexible and efficient. The Swell GraphQL API allows for precise data retrieval, while TypeScript support provides comprehensive type-checking and documentation within your IDE, improving the developer experience.
We’ll be extending the topic with our upcoming starter kits.
Up next
right to your inbox